C++标准库(STL)与泛型编程
STL 六大部件(Components)
- 容器(Containers)
- 分配器(Allocators)
- 算法(Algorithms)
- 迭代器(Iterators)
- 适配器(Adapters)
- 仿函数(Functors)

容器
STL中的容器大体分为
- 序列容器(Sequence Containers)
- 关联容器(Associative Containers)
- 无序容器(Unordered Containers)

STL设计模式
OOP(Object-Oriented Programming)和GP(Generic Programming)是STL容器设计中使用的两种设计模式。
- OOP 的目的是将数据和方法绑定在一起,例如对
std::list
容器进行排序要调用std::list::sort
方法。
- GP 的目的是将数据和方法分离开来,例如对
std::vector
容器进行排序要调用std::sort
方法。
这种不同是因为std::sort
方法内部调用了iterator
的-
运算,std::list
的iterator
没有实现-
运算符,而std::vector
的iterator
实现了-
运算符。
STL仿函数源码分析
仿函数是一类重载了 () 运算符的类,其对象可当作函数来使用,常被用做 STL 算法的参数。
STL 的所有仿函数都必须继承自基类 unary_function 或 binary_function,这两个基类定义了一系列关联类型,这些关联类型可被 STL 适配器使用。为了扩展性,我们自己写的仿函数也应当继承自这两个基类之一。
1 2 3 4 5 6 7 8 9 10 11 12
| template<class Arg, class Result> struct unary_function { typedef Arg argument_type; typedef Result result_type; };
template<class Argl, class Arg2, class Result> struct binary_function { typedef Argl first_argument_type; typedef Arg2 second_argument_type; typedef Result result_type; };
|
仿函数示例:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| template<class T> struct plus : public binary_function<T, T, T> { T operator()(const T &x, const T &y) const { return x + y; } };
template<class T> struct minus : public binary_function<T, T, T> { T operator()(const T &x, const T &y) const { return x - y; } };
template<class T> struct logical_and : public binary_function<T, T, bool> { bool operator()(const T &x, const T &y) const { return x && y; } };
template<class T> struct equal_to : public binary_function<T, T, bool> { bool operator()(const T &x, const T &y) const { return x == y; } };
template<class T> struct less : public binary_function<T, T, bool> { bool operator()(const T &x, const T &y) const { return x < y; } }
|
如果我们自定义的仿函数并没有继承自基类 unary_function 或 binary_function,这样虽然能够运行,但是不好,因为没有继承自基类的仿函数无法与 STL 其他组件(尤其是函数适配器)结合起来。
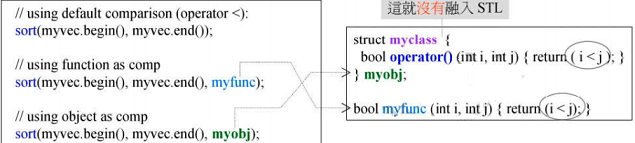